Mastering C++ Programming for Engineering Applications: A Comprehensive Guide
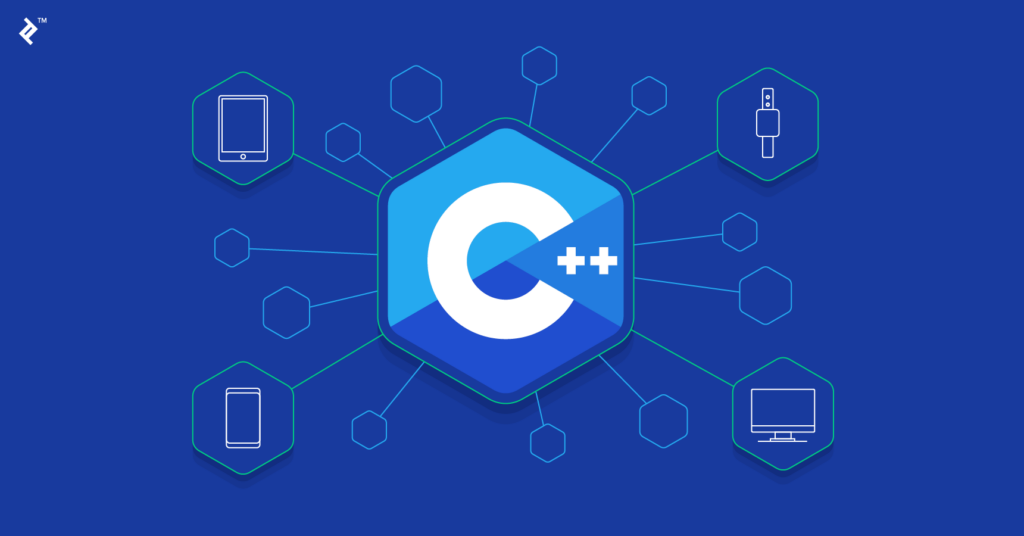
Introduction: C++ is a powerful and versatile programming language widely used in engineering for developing efficient, robust, and high-performance applications. From embedded systems and real-time control to numerical analysis and simulation, C++ offers a rich set of features and libraries that make it well-suited for engineering tasks. In this comprehensive guide, we’ll explore the intricacies of programming in C++ for engineering applications, covering everything from fundamental concepts to advanced techniques and best practices.
Section 1: Understanding C++ Basics 1.1 Introduction to C++: Get acquainted with the basics of C++ programming language, including its syntax, semantics, and key features. Understand the differences between C++ and other programming languages, such as C, Java, and Python, and why C++ is preferred for engineering applications. Explore the history and evolution of C++ and its role in software development across various domains.
1.2 Setting up the Development Environment: Before you can start programming in C++, you’ll need to set up a development environment on your computer. Choose a suitable integrated development environment (IDE) such as Visual Studio, Eclipse, or Code::Blocks, and install the necessary compiler and libraries for C++ programming. Configure the IDE to your preferences, including code formatting, syntax highlighting, and debugging tools, to streamline your development workflow.
Section 2: Mastering C++ Language Features 2.1 Data Types and Variables: Learn about the different data types available in C++, including integers, floating-point numbers, characters, and booleans, and how to declare and initialize variables of each type. Understand the concept of data type sizes, ranges, and precision, and choose appropriate data types based on the requirements of your engineering applications. Explore advanced data types such as arrays, structures, classes, and enums for organizing and manipulating data efficiently.
2.2 Control Structures and Functions: Explore control structures such as loops, conditionals, and branching statements in C++ for controlling the flow of program execution. Learn how to use if-else statements, switch-case statements, and loop constructs such as for, while, and do-while loops to implement logic and algorithms in your engineering applications. Define and call functions to encapsulate reusable code and modularize your programs for better maintainability and readability.
Section 3: Object-Oriented Programming (OOP) in C++ 3.1 Classes and Objects: Dive into the principles of object-oriented programming (OOP) in C++ and learn how to define classes and create objects to model real-world entities and behaviors. Understand the concepts of encapsulation, inheritance, and polymorphism, and how they enable modular and extensible software design in C++. Design class hierarchies and relationships to represent complex systems and components in engineering applications.
3.2 Abstraction and Encapsulation: Explore the concepts of abstraction and encapsulation in C++ and how they enable data hiding and information hiding in object-oriented design. Learn how to define class interfaces, access modifiers, and member functions to encapsulate data and behavior within classes, providing a clear separation of concerns and improving code maintainability and reusability. Use access specifiers such as public, private, and protected to control the visibility and accessibility of class members.
Section 4: Advanced C++ Techniques for Engineering Applications 4.1 Template Programming: Discover the power of template programming in C++ for creating generic algorithms and data structures that work with any data type. Learn how to define function templates and class templates to write reusable code that adapts to different types and requirements. Explore the Standard Template Library (STL) and its collection of generic algorithms, containers, and iterators for common engineering tasks such as data processing, sorting, and searching.
4.2 Multithreading and Parallel Programming: Harness the capabilities of multithreading and parallel programming in C++ to leverage modern multicore processors for improved performance and efficiency. Learn how to create and manage threads using the C++11 threading library, synchronize thread execution using mutexes, locks, and condition variables, and coordinate concurrent tasks using futures and promises. Explore parallel programming techniques such as parallel loops, tasks, and parallel algorithms for speeding up computationally intensive tasks in engineering applications.
Section 5: Libraries and Frameworks for Engineering Applications 5.1 Numerical Libraries: Explore popular numerical libraries and frameworks for C++ programming, such as Boost, Eigen, and Armadillo, that provide powerful tools for numerical computation, linear algebra, and optimization. Learn how to use these libraries to solve linear systems, perform matrix operations, compute eigenvalues and eigenvectors, and optimize algorithms for performance and accuracy in engineering simulations and analysis.
5.2 Graphics and Visualization Libraries: Discover graphics and visualization libraries for C++ programming, such as OpenGL, Vulkan, and DirectX, that enable the creation of interactive 2D and 3D graphics applications for engineering visualization and simulation. Learn how to use these libraries to render complex scenes, create interactive user interfaces, and visualize engineering data in real-time for analysis, presentation, and design validation.
Conclusion: C++ programming offers engineers a powerful and flexible toolset for developing sophisticated engineering applications across various domains. By mastering the fundamentals of C++ programming and exploring advanced techniques and libraries tailored for engineering tasks, engineers can create robust, efficient, and scalable software solutions that meet the demands of modern engineering challenges. With practice, experimentation, and continuous learning, engineers can leverage the full potential of C++ to innovate, optimize, and solve complex engineering problems with confidence and creativity.