Mastering the Python subprocess Module: A Comprehensive Guide
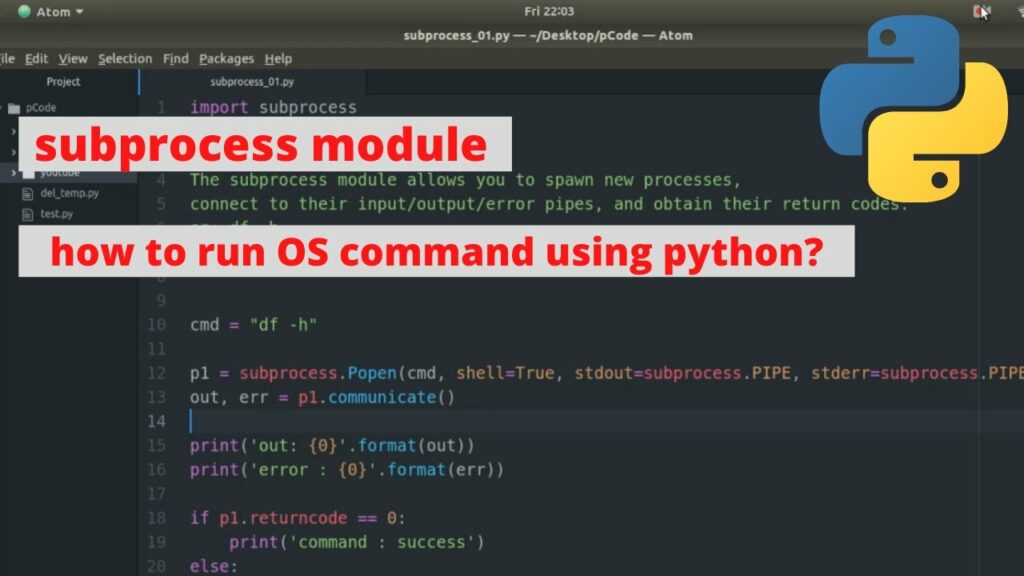
Introduction to subprocess
The subprocess
module in Python provides a way to spawn new processes, connect to their input/output/error pipes, and obtain their return codes. It’s a versatile tool for interacting with external programs and automating tasks.
Basic Usage: subprocess.run()
The run()
function is a high-level interface for calling external commands and capturing their output.
import subprocess
result = subprocess.run(["ls", "-la"], capture_output=True, text=True)
if result.returncode == 0:
print(result.stdout)
else:
print(result.stderr)
capture_output=True
: Captures both stdout and stderr.text=True
: Decodes the output as text.
The subprocess.Popen
Class
For more granular control over the subprocess, use the Popen
class:
import subprocess
process = subprocess.Popen(["ls", "-la"], stdout=subprocess.PIPE)
output, error = process.communicate()
if process.returncode == 0:
print(output.decode())
else:
print(error.decode())
stdout=subprocess.PIPE
: Captures the standard output of the subprocess.communicate()
waits for the process to terminate and returns its stdout and stderr.
Understanding Arguments
The args
argument to subprocess.run()
or subprocess.Popen()
can be a sequence of program arguments or a single string.
# Sequence of arguments
subprocess.run(["python", "my_script.py", "arg1", "arg2"])
# Single string (shell-like)
subprocess.run("python my_script.py arg1 arg2", shell=True)
Caution: Using shell=True
can be a security risk, as it allows arbitrary shell commands to be executed. Use it with caution and only when necessary.
Input and Output Redirection
- Standard Input:Python
process = subprocess.Popen(["my_program"], stdin=subprocess.PIPE) process.stdin.write(b"some input data") process.stdin.close()
- Standard Output and Error:Python
process = subprocess.Popen(["my_program"], stdout=subprocess.PIPE, stderr=subprocess.PIPE) output, error = process.communicate()
Process Control
- Waiting for Process Termination:Python
process.wait()
- Checking Process Status:Python
returncode = process.poll() if returncode is not None: print("Process has finished with return code:", returncode)
- Terminating a Process:Python
process.terminate()
Error Handling
It’s essential to handle potential errors when working with subprocesses:
import subprocess
try:
result = subprocess.run(["nonexistent_command"], capture_output=True, text=True, check=True)
except subprocess.CalledProcessError as e:
print(f"Command failed with return code {e.returncode}")
print(e.output)
The check=True
argument raises a subprocess.CalledProcessError
if the return code is non-zero.
Advanced Usage
- Pipes: Connect the output of one process to the input of another:Python
p1 = subprocess.Popen(["ls", "-la"], stdout=subprocess.PIPE) p2 = subprocess.Popen(["grep", "python"], stdin=p1.stdout, stdout=subprocess.PIPE) p1.stdout.close() output, error = p2.communicate()
- Environment Variables: Set environment variables for the subprocess:Python
env = os.environ.copy() env["MY_VARIABLE"] = "value" subprocess.run(["my_program"], env=env)
- Working Directory: Change the working directory for the subprocess:Python
subprocess.run(["ls"], cwd="/tmp")
Conclusion
The subprocess
module is a powerful tool for interacting with external programs and automating tasks in Python. By understanding its core concepts and features, you can effectively leverage its capabilities to build robust and efficient applications.