Mastering the Python Logging Module: A Comprehensive Guide
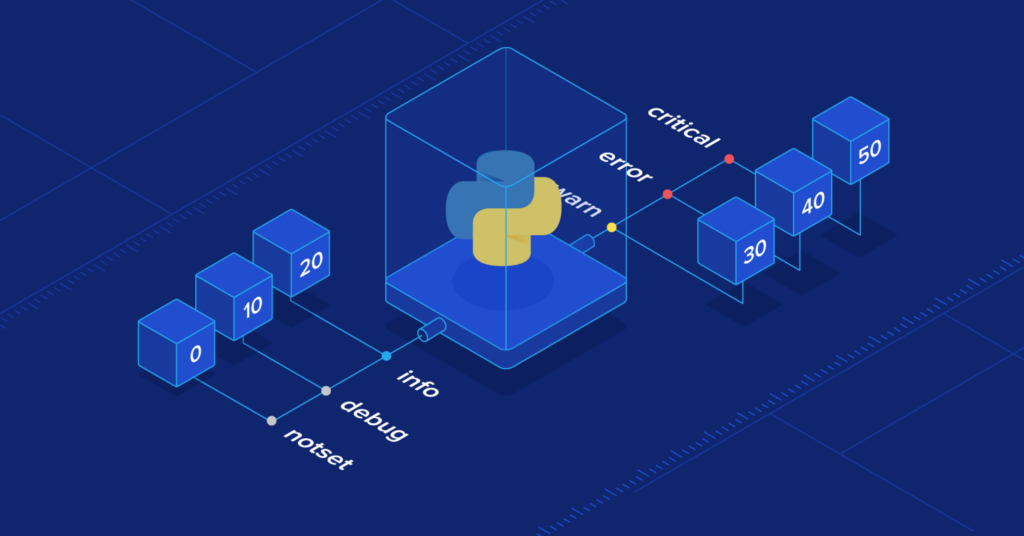
Introduction to Python Logging
The Python logging
module is a versatile and flexible tool for recording log messages in your applications. It provides a robust framework for capturing, filtering, and routing log messages to various destinations, such as the console, files, or network sockets. Effective logging is essential for debugging, monitoring, and troubleshooting your applications.
Basic Logging
To start logging, import the logging
module:
import logging
You can use the logging
module directly to log messages at different levels:
import logging
logging.debug("This is a debug message")
logging.info("This is an informational message")
logging.warning("This is a warning message")
logging.error("This is an error message")
logging.critical("This is a critical message")
By default, only messages with a level of WARNING
or higher are displayed. You can change this behavior using the basicConfig
function.
Configuring the Logging Module
To customize the logging behavior, you can use the basicConfig
function:
import logging
logging.basicConfig(level=logging.DEBUG,
format='%(asctime)s - %(name)s - %(levelname)s - %(message)s',
datefmt='%d-%m-%y %H:%M:%S')
This configuration sets the logging level to DEBUG
, defines a format for the log messages, and specifies a date format.
Log Levels
The logging module defines several log levels:
DEBUG
: Detailed information, typically used for debugging.INFO
: Confirm that things are working as expected.WARNING
: An indication that something unexpected happened, or indicative of some problem in the near future.ERROR
: Due to a more serious problem, the software has not been able to perform some function.CRITICAL
: A serious error, indicating that the program itself may be unable to continue.
Loggers, Handlers, and Formatters
For more complex logging scenarios, you can create custom loggers, handlers, and formatters.
- Loggers: Represent a category or subsystem used to track messages.
- Handlers: Send log messages to specific destinations (e.g., console, file, network).
- Formatters: Control the appearance of log messages.
import logging
logger = logging.getLogger(__name__)
logger.setLevel(logging.DEBUG)
# Create a file handler
file_handler = logging.FileHandler('my_log.log')
file_handler.setLevel(logging.INFO)
# Create a console handler
console_handler = logging.StreamHandler()
console_handler.setLevel(logging.WARNING)
# Create a formatter
formatter = logging.Formatter('%(asctime)s - %(name)s - %(levelname)s - %(message)s')
# Add formatter to handlers
file_handler.setFormatter(formatter)
console_handler.setFormatter(formatter)
# Add handlers to logger
logger.addHandler(file_handler)
logger.addHandler(console_handler)
# Log messages
logger.debug('Debug message')
logger.info('Info message')
logger.warning('Warning message')
logger.error('Error message')
logger.critical('Critical message')
Advanced Logging Features
- Filters: Control which log records are passed to handlers.
- Logger Hierarchy: Create hierarchical loggers for organizing log messages.
- Custom Handlers: Create custom handlers for specific output formats or destinations.
- Log Rotation: Automatically rotate log files to manage disk space.
- Performance Optimization: Consider performance implications when configuring logging.
Best Practices for Logging
- Use informative log messages.
- Avoid logging sensitive information.
- Use different log levels appropriately.
- Configure logging for different environments (development, testing, production).
- Regularly review and analyze log files.
Conclusion
The Python logging module provides a powerful and flexible way to manage log messages in your applications. By understanding its core components and best practices, you can effectively use logging to improve your code’s reliability, maintainability, and troubleshooting capabilities.