Mastering Exception Handling in Python: A Comprehensive Guide
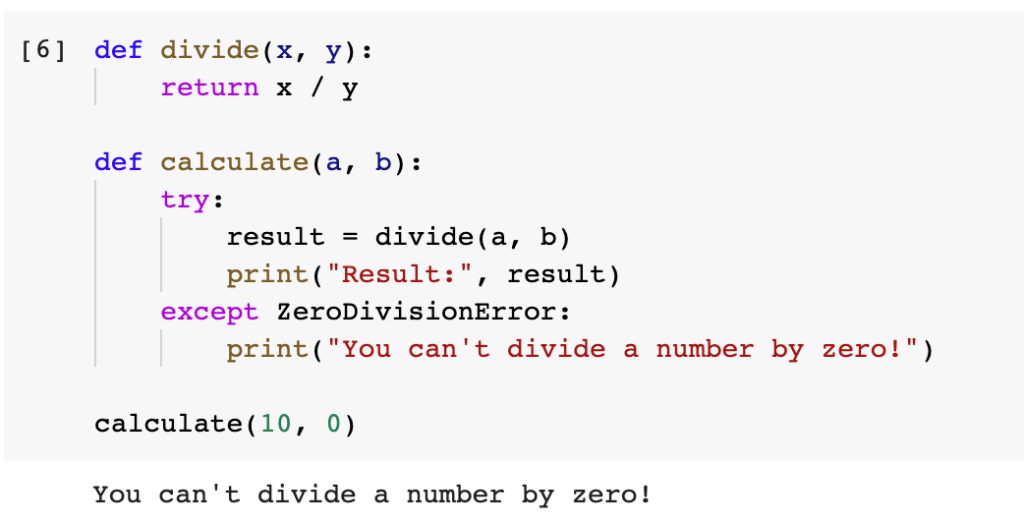
Understanding Exceptions
In Python, exceptions are errors that occur during program execution. When an exception arises, the normal flow of the program is interrupted unless handled appropriately. Understanding and handling exceptions is crucial for writing robust and reliable code.
The try-except
Block
The fundamental construct for handling exceptions is the try-except
block.
try:
# Code that might raise an exception
except ExceptionType:
# Code to handle the exception
try
block: Contains code that might raise an exception.except
block: Contains code to handle the specific exception type.
def divide(x, y):
try:
result = x / y
print("Result:", result)
except ZeroDivisionError:
print("Error: Division by zero")
divide(10, 2) # Output: Result: 5.0
divide(10, 0) # Output: Error: Division by zero
Handling Multiple Exceptions
You can handle multiple exception types in a single try-except
block:
try:
# Code that might raise different exceptions
except ExceptionType1:
# Handle ExceptionType1
except ExceptionType2:
# Handle ExceptionType2
except:
# Handle other exceptions
The last except
clause without a specific exception type is a catch-all for any exception not explicitly handled. It’s generally discouraged as it can mask unexpected errors.
The else
Clause
The else
clause can be used to specify code that should run if no exceptions occur:
try:
# Code that might raise an exception
except ExceptionType:
# Handle exception
else:
# Code to run if no exception occurs
The finally
Clause
The finally
clause ensures that certain code is executed regardless of whether an exception occurs or not:
try:
# Code that might raise an exception
except ExceptionType:
# Handle exception
else:
# Code to run if no exception occurs
finally:
# Code that always runs
This is often used for cleanup actions, such as closing files or database connections.
Raising Exceptions
You can raise exceptions explicitly using the raise
keyword:
def divide(x, y):
if y == 0:
raise ValueError("Cannot divide by zero")
else:
return x / y
Custom Exceptions
You can create custom exception classes by inheriting from the Exception
class:
class CustomError(Exception):
pass
def my_function():
raise CustomError("This is a custom error")
Best Practices for Exception Handling
- Be specific about the exceptions you catch.
- Avoid bare
except
clauses. - Use
try-except
blocks judiciously. - Provide informative error messages.
- Test your exception handling thoroughly.
- Consider using context managers for resource management.
- Document potential exceptions in your code.
Common Built-in Exceptions
ZeroDivisionError
: Division by zeroValueError
: Invalid argument type or valueTypeError
: Type mismatchIndexError
: Index out of rangeKeyError
: Key not found in dictionaryFileNotFoundError
: File not foundIOError
: Input/output operation failed
Advanced Exception Handling
- Exception chaining: Linking exceptions for debugging.
- Exception hierarchies: Creating custom exception hierarchies.
- Context managers: Using
with
statements for resource management. - Logging exceptions: Recording exceptions for analysis.
- Assertions: Using
assert
statements for testing assumptions.
Conclusion
Effective exception handling is essential for writing robust and maintainable Python code. By understanding the core concepts and best practices, you can create applications that gracefully handle unexpected errors and provide informative feedback to users.