How to Read and Write Files in Python: A Comprehensive Guide
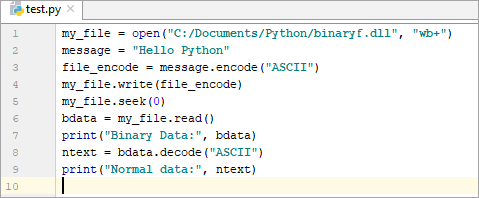
Introduction to File Handling
File handling is a fundamental aspect of programming that involves interacting with data stored on persistent storage devices. Python provides a straightforward and efficient way to read, write, and manipulate files. This comprehensive guide will explore various file operations and best practices.
Opening and Closing Files
Before performing any file operations, you need to open the file. The open()
function is used for this purpose. It returns a file object, which represents the opened file.
file = open("filename.txt", "r") # Open for reading
The second argument, the mode, specifies how the file will be used:
- ‘r’: Read mode (default).
- ‘w’: Write mode (creates a new file or truncates an existing one).
- ‘a’: Append mode (appends to the end of an existing file or creates a new one).
- ‘r+’: Read and write mode.
- ‘w+’: Read and write mode (truncates the file first).
- ‘a+’: Read and append mode.
It’s crucial to close the file after use to release system resources.
file.close()
Reading from Files
Python offers several methods to read data from files.
Reading the Entire File
file = open("filename.txt", "r")
content = file.read()
print(content)
file.close()
Reading Line by Line
file = open("filename.txt", "r")
for line in file:
print(line, end="")
file.close()
Reading Specific Number of Characters
file = open("filename.txt", "r")
content = file.read(10) # Read the first 10 characters
print(content)
file.close()
Writing to Files
To write data to a file, use the write()
method.
file = open("output.txt", "w")
file.write("This is some text.\n")
file.write("This is another line.\n")
file.close()
Appending to Files
Use the ‘a’ mode to append data to an existing file.
file = open("output.txt", "a")
file.write("Appended text.\n")
file.close()
Working with Binary Files
For binary files, use the ‘rb’ (read binary), ‘wb’ (write binary), and ‘ab’ (append binary) modes.
import pickle
# Writing to a binary file
data = [1, 2, 3, "hello"]
with open("data.bin", "wb") as file:
pickle.dump(data, file)
# Reading from a binary file
with open("data.bin", "rb") as file:
loaded_data = pickle.load(file)
print(loaded_data)
Context Managers and the with
Statement
To ensure proper file closing even in case of exceptions, use the with
statement:
with open("filename.txt", "r") as file:
# File operations here
# The file will be closed automatically at the end of the block
File Modes and Permissions
Understanding file modes and permissions is crucial for secure file handling.
- Read-only: Only allows reading the file.
- Write-only: Creates a new file or truncates an existing one for writing.
- Read and write: Allows both reading and writing to the file.
- Append: Appends data to the end of the file.
- Binary: Handles binary data.
- Text: Handles text data.
Error Handling
It’s essential to handle potential file-related errors using try-except
blocks:
try:
with open("filename.txt", "r") as file:
# File operations
except FileNotFoundError:
print("File not found.")
except PermissionError:
print("Permission denied.")
Advanced File Operations
- File objects: Explore attributes like
name
,mode
,closed
, and methods likeseek
,tell
,flush
. - File-like objects: Learn about objects that mimic file behavior (e.g.,
StringIO
,BytesIO
). - OS module: Use functions like
os.path.exists
,os.path.isfile
,os.path.isdir
,os.rename
, andos.remove
for file and directory operations. - shutil module: Utilize functions for high-level file operations like copying, moving, and deleting files and directories.
Best Practices
- Use descriptive file names.
- Close files explicitly or use
with
statements. - Handle exceptions gracefully.
- Optimize file I/O for performance.
- Consider using compression for large files.
- Be mindful of file permissions and security.
Conclusion
Python provides a powerful and flexible set of tools for file handling. By understanding the fundamentals and best practices, you can effectively manage file operations in your Python applications.