How to Install and Use Pip: A Comprehensive Guide
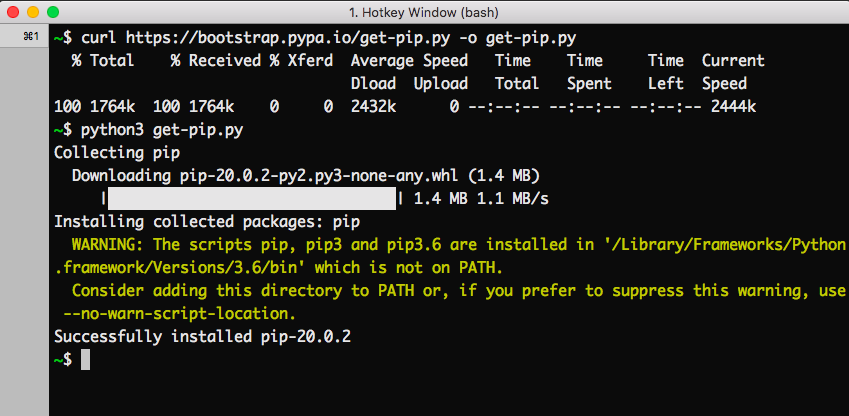
Introduction
Pip is the package installer for Python and is a fundamental tool for managing Python packages and libraries. It simplifies the process of installing, updating, and removing Python packages from the Python Package Index (PyPI) and other repositories. This guide provides a comprehensive overview of how to install and use pip effectively, from initial setup to advanced usage.
Table of Contents
- What is Pip?
- Installing Pip
- Installing Pip with Python Installation
- Installing Pip Separately
- Installing Pip on Different Operating Systems
- Using Pip
- Basic Commands
- Installing Packages
- Upgrading and Uninstalling Packages
- Managing Dependencies
- Configuring Pip
- Using a Configuration File
- Setting Up Environment Variables
- Advanced Pip Usage
- Using Pip with Virtual Environments
- Installing Packages from a Requirements File
- Installing Packages from Git Repositories
- Troubleshooting Common Issues
- Best Practices for Using Pip
- Conclusion
What is Pip?
Pip is the package installer for Python, designed to manage libraries and dependencies in Python projects. It allows you to download, install, and manage packages from PyPI and other sources. Pip is widely used in the Python community due to its ease of use and ability to handle complex dependencies.
Key features of pip include:
- Package Installation: Download and install packages from PyPI or other repositories.
- Dependency Management: Automatically resolve and install package dependencies.
- Version Control: Install specific versions of packages or upgrade to the latest version.
- Requirements Files: Install packages listed in a
requirements.txt
file for project consistency.
Installing Pip
Pip is included by default with Python installations starting from Python 3.4. However, you may need to install or update it separately if it’s not available on your system.
Installing Pip with Python Installation
If you have Python 3.4 or later, pip should be included with your Python installation. You can check if pip is installed by running the following command:
pip --version
If pip is not installed, you can install it using the ensurepip
module:
python -m ensurepip --upgrade
Installing Pip Separately
If pip is not included with your Python installation, you can install it manually using the get-pip.py
script.
- Download
get-pip.py
:Download the
get-pip.py
script from the official repository. - Run
get-pip.py
:Open a terminal or command prompt and navigate to the directory where
get-pip.py
is saved. Run the following command:shpython get-pip.py
This will install pip and its dependencies.
Installing Pip on Different Operating Systems
Pip installation procedures may vary slightly depending on your operating system.
On Windows
- Ensure Python is Installed: Download and install Python from the official website. During installation, make sure to check the box that says “Add Python to PATH”.
- Verify Pip Installation: Open Command Prompt and check if pip is installed:sh
pip --version
If pip is not installed, you can follow the manual installation steps provided above.
On macOS
- Ensure Python is Installed: Python is pre-installed on macOS, but you can install the latest version using Homebrew:sh
brew install python
- Verify Pip Installation: Open Terminal and check if pip is installed:sh
pip3 --version
Note that on macOS, pip is often referred to as
pip3
for Python 3.x.
On Linux
- Ensure Python is Installed: Python is typically pre-installed on Linux distributions. To install Python and pip, use your distribution’s package manager:
- Debian/Ubuntu:sh
sudo apt update
sudo apt install python3 python3-pip
- Fedora:sh
sudo dnf install python3 python3-pip
- Arch Linux:sh
sudo pacman -S python python-pip
- Debian/Ubuntu:
- Verify Pip Installation: Open Terminal and check if pip is installed:sh
pip3 --version
Using Pip
Pip provides a variety of commands to manage Python packages. Understanding these commands will help you effectively manage your Python environment.
Basic Commands
Here are some basic pip commands and their functions:
- Check Pip Version:sh
pip --version
- Upgrade Pip:sh
pip install --upgrade pip
Installing Packages
To install a package using pip, use the following command:
pip install package_name
For example, to install the requests
library:
pip install requests
Installing Specific Versions
You can specify a version of a package to install:
pip install package_name==1.0.0
Installing Multiple Packages
To install multiple packages at once, list them separated by spaces:
pip install numpy pandas scipy
Upgrading and Uninstalling Packages
To upgrade an installed package to the latest version:
pip install --upgrade package_name
To uninstall a package:
pip uninstall package_name
You will be prompted to confirm the uninstallation.
Managing Dependencies
Pip handles dependencies automatically by installing any required packages for the package you are installing. You can view the installed packages and their dependencies with:
pip show package_name
Configuring Pip
Pip can be customized using configuration files and environment variables to tailor its behavior to your needs.
Using a Configuration File
Pip supports configuration files that allow you to set various options and preferences. Configuration files can be placed in several locations:
- User-Level Configuration:
~/.pip/pip.conf
(Linux/macOS) or%APPDATA%\pip\pip.ini
(Windows) - Global Configuration:
/etc/pip.conf
(Linux/macOS) orC:\ProgramData\pip\pip.ini
(Windows)
Sample configuration (pip.conf
):
[global]
timeout = 60
index-url = https://pypi.org/simple
trusted-host = pypi.org
Setting Up Environment Variables
You can set environment variables to configure pip’s behavior:
- PIP_INDEX_URL: Specify the base URL of the Python Package Index (PyPI).sh
export PIP_INDEX_URL=https://pypi.org/simple
- PIP_TRUSTED_HOST: Specify a trusted host.sh
export PIP_TRUSTED_HOST=pypi.org
Advanced Pip Usage
Pip offers advanced features for managing packages, including working with virtual environments, requirements files, and Git repositories.
Using Pip with Virtual Environments
Virtual environments allow you to create isolated Python environments with their own packages and dependencies. This helps avoid conflicts between project dependencies.
- Create a Virtual Environment:sh
python -m venv myenv
- Activate the Virtual Environment:
- Windows:sh
myenv\Scripts\activate
- macOS/Linux:sh
source myenv/bin/activate
- Windows:
- Install Packages in the Virtual Environment:
With the virtual environment activated, use pip to install packages:
shpip install package_name
- Deactivate the Virtual Environment:sh
deactivate
Installing Packages from a Requirements File
A requirements.txt
file lists packages and their versions for your project. You can use this file to install all the dependencies at once.
- Create a
requirements.txt
File:Example
requirements.txt
:txtnumpy==1.21.2
pandas==1.3.3
- Install Packages from
requirements.txt
:shpip install -r requirements.txt
Installing Packages from Git Repositories
Pip can install packages directly from Git repositories.
- Install from a Git Repository:sh
pip install git+https://github.com/user/repo.git
- Install a Specific Branch or Tag:sh
pip install git+https://github.com/user/repo.git@branch_name
Troubleshooting Common Issues
While using pip, you might encounter some common issues. Here are solutions to help you troubleshoot:
Common Issues
- Permission Errors:
If you encounter permission errors, try using the
--user
flag to install packages locally for the current user:shpip install --user package_name
- Package Not Found:
Ensure you have the correct package name and version. Verify your network connection and PyPI accessibility.
- Conflicting Dependencies:
If you have conflicting dependencies, consider using a virtual environment to isolate project-specific packages.
Debugging Tips
- Check Pip Logs: Use the
-v
(verbose) option to get more detailed output from pip:shpip install -v package_name
- Use the
--no-cache-dir
Option: If you encounter issues with cached files, use the--no-cache-dir
option:shpip install --no-cache-dir package_name
- Upgrade Pip: Ensure you are using the latest version of pip:sh
pip install --upgrade pip
Best Practices for Using Pip
To ensure smooth package management and project stability, follow these best practices:
Keep Pip Updated
Regularly update pip to benefit from the latest features and bug fixes:
pip install --upgrade pip
Use Virtual Environments
Create and use virtual environments for each project to avoid dependency conflicts and manage package versions effectively.
Manage Dependencies with Requirements Files
Use requirements.txt
files to document and manage project dependencies. This ensures consistent environments across different systems and setups.
Check Package Compatibility
Before installing or upgrading packages, check for compatibility issues and review release notes or documentation.
Use Trusted Sources
Only install packages from trusted sources and repositories. Be cautious of packages from unknown or unverified sources.
Conclusion
Pip is an essential tool for Python developers, providing a straightforward way to manage packages and dependencies. By following this comprehensive guide, you have learned how to install and use pip, configure it to suit your needs, and troubleshoot common issues. Embrace best practices to ensure effective package management and maintain a stable Python environment. Happy coding and managing your Python packages with pip!